It will come as a shock to absolutely no one that I struggle with live coding challenges. Many people struggle with doing work when they are being watched, and there are a number of scientific theories and descriptors as to why. It took me a couple of live coding challenges to realize what was going on but once I did the answer was mercifully simple: practice. Practice until I achieve a level of reflexive fluency.
With human languages, spoken languages such as Japanese or Italian, I would have a chapter in a textbook to study. I would have phrases and vocabulary to memorize and use in sentences, and I might find an environment where I could listen and repeat them in a conversational manner. I might even seek out a partner to practice with. Computer languages aren’t taught in the same established textbook format as spoken languages, but there is still a vocabulary of built-in methods one can learn, and a syntax, and a set of basic sentences beginning with a “Hello World!” function. So when I had trouble with my React code challenge, I set up my practice environment where I could repeat the basic React phrases, and I started repeating.
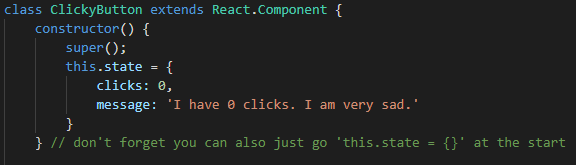
One of the first things I had trouble with in the React live-code challenge was remembering that state exists. So, creating components that used and altered state was the first thing I needed to do. In this case, making a button that shows how many clicks I’ve made. Overall I wrote between 10 and 15 little React files that used and altered state, but ClickyButton was the first one.
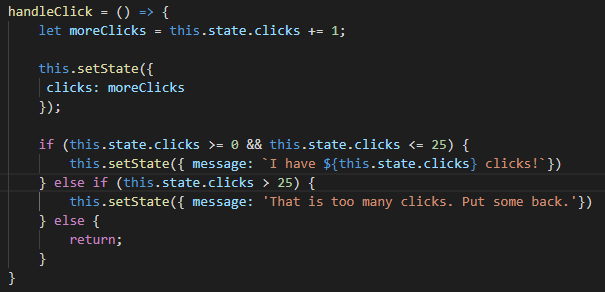
After setting state, I had to make an event handler. This was more familiar from older attempts at learning and using JavaScript, but using an event handler to modify state was new, so I needed to practice it. Memorize it, as one might memorize a “Nice to meet you” or a “Where is the restaurant” in a basic dialogue exercise for a human language. For the first step, I created the incrementor and then used setState to update the state.
And then, to make it a little more complex and decorative, I added an if/then/else block. This was both to add a bit of complexity to the component and to remind myself of the format of an if/then/else block in JavaScript. I haven’t done as much JavaScript over the years as I have done CSS and HTML, and I took better to Ruby in bootcamp, so a little reminder couldn’t hurt.
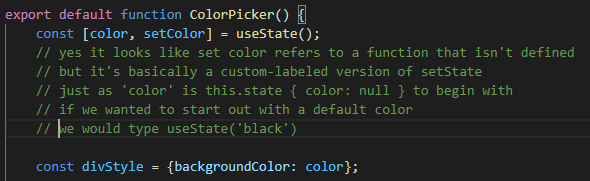
I didn’t learn about hooks in bootcamp, but I did learn about them in CodeAcademy, so I did some practice with those too. And it would help me practice thinking of React as using state. For this exercise I had a single useState hook to change the color of a div and several buttons that, when clicked, would call that setColor function. (I also, as you see, left myself comments to explain how it would work because I knew it would take me a few minutes when I went back and looked to remember how it all hangs together.)
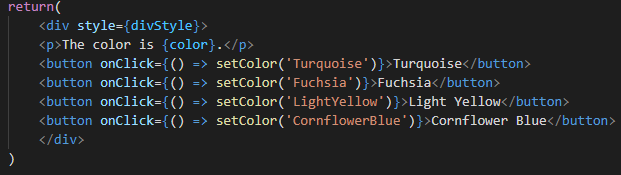
And then the second part of hook practice: using the setColor function. Each button changes the color in the div according to the divStyle assignment in the previous graphic, as explained above.
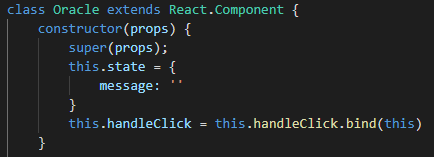
Another thing I definitely wanted to practice and make instinctive was props. Similar to state, it was a concept that hadn’t yet taken hold in the back of my mind, so I made a few components that used props. In this case, I would hard-code the props into the main app component because the point (at this time) wasn’t to learn how to pass around the props, but how to pass down the props and use them in the component.
I also wanted to practice binding methods to their specific instances. I prefer using arrow functions, but it’s always good to have a few methods in your tool-belt for doing different things; in this case I used the bind for the handleClick method.
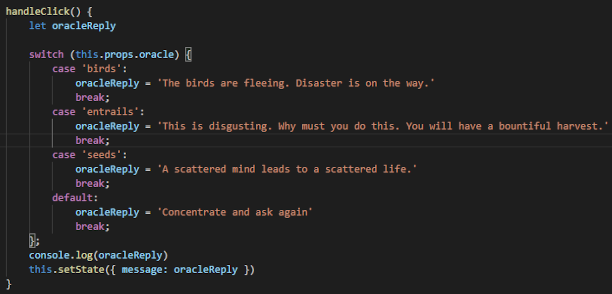
For the handler method I used a switch/case statement, because I wanted to have multiple options depending on what the props were. I declared the variable for the result at the top, and then set it to different strings for each case. Because this was a bit complex involving some modification to the main app component, I included a console-log to track my progress.
This is not, by any means, a complete list of all the aspects of React I practiced. I mixed and matched all of these code fragments to create small, simple apps that changed text, changed colors, responded to clicks. As with practicing for dialogue challenges in the various human languages I’ve studied, it helps me to keep a space, a git repository, to practice the basic “phrases” of React and increase my fluency in the framework so that when my next code challenge appears, it comes instinctively and without more than an initial moment of freeze.